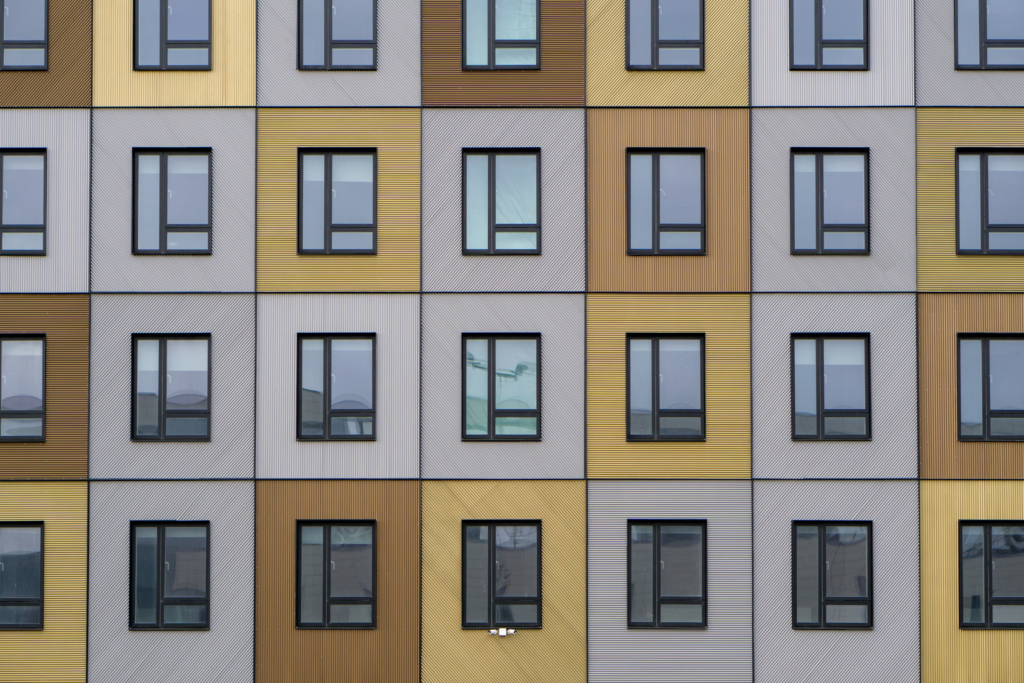
In web applications, sharing data between different tabs can be a challenge. Imagine you’re working on a Single Page Application (SPA) and need to share information between the /process/emailing/
tab and the /process
tab. Reloading the entire page disrupts the user experience, so a more elegant solution is required. In this rest of this article we will learn how to effectively communicate between browser tabs using the Broadcast Channel API.
The Broadcast Channel API: A Simple yet Powerful Solution
This is where the Broadcast Channel API steps in. This powerful tool allows to seamlessly communicate between browser tabs within the same web application. It works by broadcasting messages to all channels with the same name, enabling data exchange without page reloads.
Creating a Broadcast Channel
Let’s get started! Here’s how to create a broadcast channel:
const channel = new BroadcastChannel('oauth')
We assign the channel a name, in this case, “oauth.” This name will be used later to listen for messages on this specific channel.
Sending Messages
Once the channel is created, you can send messages using the postMessage
method:
channel.postMessage(data)
The data
parameter can be any object you want to share.
The Broadcast Channel API supports a wide range of data types, including:
- Primitive types (except symbols)
- Arrays
- Object literals
- String, Date, RegExp objects
- Blob, File, FileList objects
- ArrayBuffer, ArrayBufferView objects
- FormData objects
- ImageData objects
- Map and Set objects
Listening for Messages
To receive messages on the same channel in other parts of your application, simply create a new channel with the same name and use the onmessage
event handler:
const channel = new BroadcastChannel('oauth')
channel.onmessage = (e) => {
// Handle the received data (e.data) here
}
The e.data
property within the onmessage
function holds the data sent through the channel.
Closing the Channel
When you’re finished using a channel, you can close it to prevent further message reception:
channel.close()
Alternative Approaches
While the Broadcast Channel API is a fantastic solution, there are other methods for achieving inter-tab communication, such as the SharedWorker API or Local Storage. However, the Broadcast Channel API offers a more intuitive and straightforward approach in many scenarios.
Do you have any other preferred methods for communicating between browser tabs? Share your thoughts in the comments!